🌟 Excited to dive into some Machine Learning magic? 🌟
Hello, aspiring data wizards! Are you ready to level up your Machine Learning skills? Whether you're just starting or a seasoned pro, there's always something new to learn in this ever-evolving field.
Today, let's unravel the mysteries of Machine Learning together. We're diving deep into a master-level question to flex those neural networks and sharpen our algorithms. Buckle up and get ready to code your way through this challenge!
🚀 Question: Building a Custom Neural Network 🚀
You've been tasked with building a custom neural network from scratch for a binary classification problem. Your network should have one hidden layer and use the sigmoid activation function for the hidden layer and the output layer. You also need to implement forward and backward propagation functions, as well as update the network's parameters using gradient descent.
Your network should be flexible enough to handle varying input sizes and should include methods for training and predicting.
Ready to tackle this challenge head-on? Let's break it down step by step!
🔍 Solution: Crafting the Neural Network 🔍
```python
import numpy as np
class CustomNeuralNetwork:
def __init__(self, input_size, hidden_size, output_size):
self.input_size = input_size
self.hidden_size = hidden_size
self.output_size = output_size
self.weights_input_hidden = np.random.randn(self.input_size, self.hidden_size)
self.bias_input_hidden = np.zeros((1, self.hidden_size))
self.weights_hidden_output = np.random.randn(self.hidden_size, self.output_size)
self.bias_hidden_output = np.zeros((1, self.output_size))
def sigmoid(self, x):
return 1 / (1 + np.exp(-x))
def forward_propagation(self, X):
self.hidden_layer_input = np.dot(X, self.weights_input_hidden) + self.bias_input_hidden
self.hidden_layer_output = self.sigmoid(self.hidden_layer_input)
self.output_layer_input = np.dot(self.hidden_layer_output, self.weights_hidden_output) + self.bias_hidden_output
self.output = self.sigmoid(self.output_layer_input)
return self.output
def backward_propagation(self, X, y, learning_rate):
error = y - self.output
output_delta = error * (self.output * (1 - self.output))
hidden_error = np.dot(output_delta, self.weights_hidden_output.T)
hidden_delta = hidden_error * (self.hidden_layer_output * (1 - self.hidden_layer_output))
self.weights_hidden_output += np.dot(self.hidden_layer_output.T, output_delta) * learning_rate
self.bias_hidden_output += np.sum(output_delta, axis=0, keepdims=True) * learning_rate
self.weights_input_hidden += np.dot(X.T, hidden_delta) * learning_rate
self.bias_input_hidden += np.sum(hidden_delta, axis=0, keepdims=True) * learning_rate
def train(self, X, y, epochs, learning_rate):
for epoch in range(epochs):
output = self.forward_propagation(X)
self.backward_propagation(X, y, learning_rate)
def predict(self, X):
return self.forward_propagation(X)
# Example usage:
# input_size = 2, hidden_size = 3, output_size = 1
# nn = CustomNeuralNetwork(2, 3, 1)
# X_train = np.array([[0, 0], [0, 1], [1, 0], [1, 1]])
# y_train = np.array([[0], [1], [1], [0]])
# nn.train(X_train, y_train, epochs=1000, learning_rate=0.1)
# predictions = nn.predict(X_train)
# print(predictions)
```
There you have it! A custom neural network implementation ready to tackle your binary classification challenges. Feel free to tweak the architecture, play with hyperparameters, and experiment with different datasets to supercharge your Machine Learning journey.
🔑 Key Takeaways 🔑
- Building a neural network from scratch empowers you to understand the inner workings of deep learning algorithms.
- Implementing forward and backward propagation is crucial for training your network to make accurate predictions.
- Experimentation and iteration are key to mastering Machine Learning. Don't be afraid to try new approaches and learn from your results.
That's a wrap for today's Machine Learning adventure! Remember, the journey to mastery is filled with challenges, but with dedication and persistence, you'll unlock a world of possibilities. Stay curious, keep coding, and never stop learning!
Drop a comment below if you found this post helpful or if you have any questions. Happy coding, and may your algorithms always converge! 🚀✨ If you need help with machine learning assignment, Visit Now at https://www.programminghomewor....khelp.com/machine-le
#helpwithmachinelearningassignment #machinelearningassignmenthelp #machinelearningassignment #programmingassignment #programmingassignmenthelp #education #students #university #college #assignmenthelp #sample #question #answer
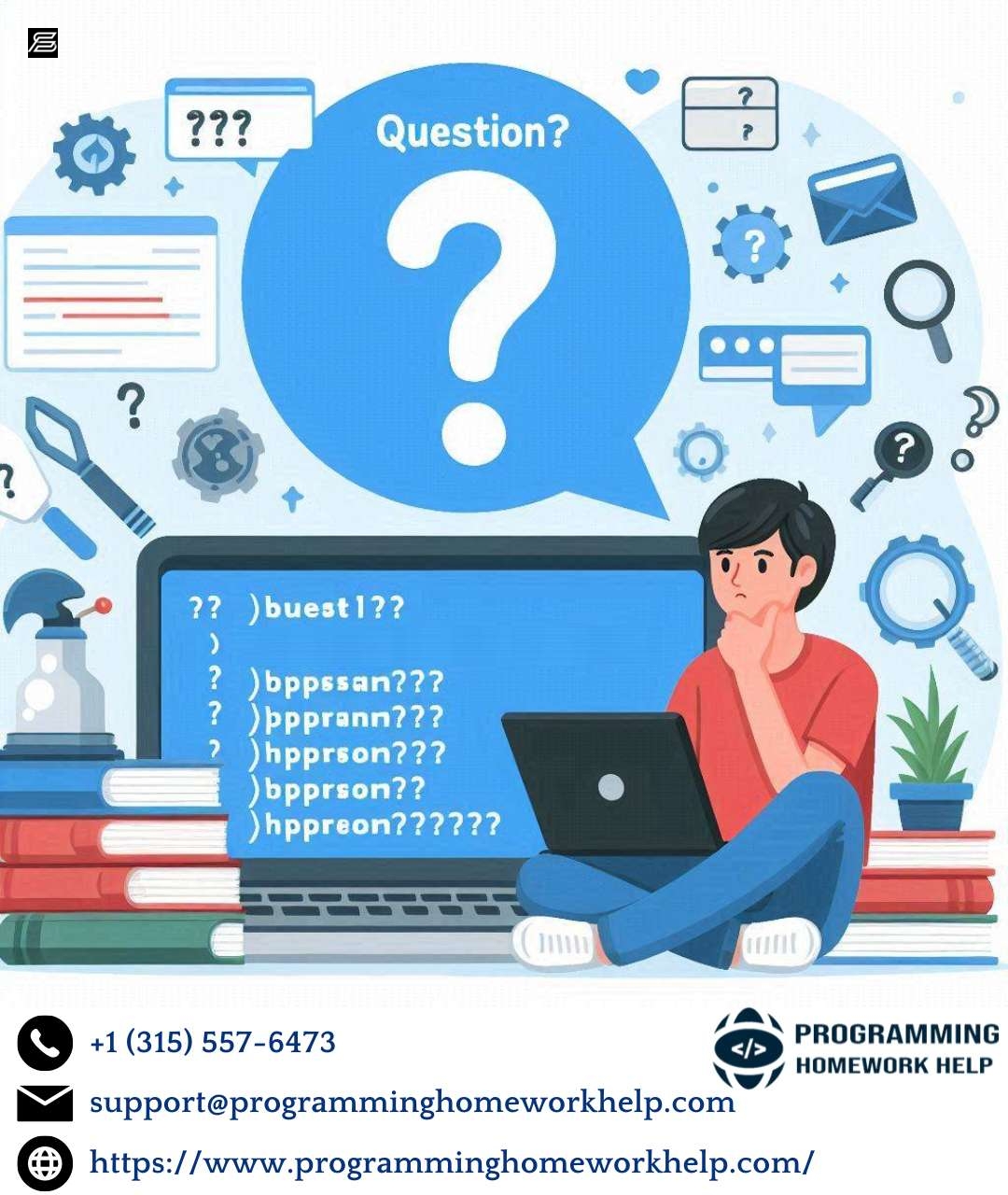